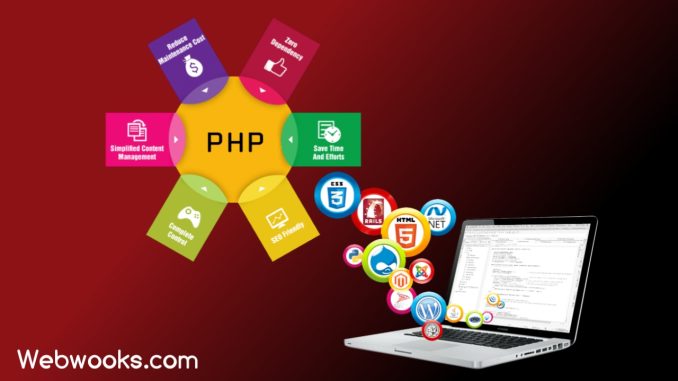
What is most important when designing a PHP website for beginners is an understanding of these basic fundamentals: HTML for the structure, CSS for the style, JavaScript for interaction, and PHP for functionality on the server-side. Here is a systematic approach to creating a simple PHP website, based on the key elements a beginner first needs to look into.
1. Setting Up Your Development Environment
Before you start coding, it is a good idea to run a local development environment on your machine. A typical setup includes:
XAMPP/WAMP/MAMP: These are application packages that provide Apache, which is a software for web servers, MySQL for database purposes, and PHP. Among them, XAMPP is very famous because of its easy interface and working on almost all operating systems.
Code Editor: The student will utilize a code editor like Visual Studio Code, Sublime text, or PHPStorm to write PHP and related code.
Once installed, make sure the servers of both PHP and MySQL are ON to test your website locally.
2. Create a Folder for Your Project
Once you have your environment configured, create a directory within the server directory- for example, if you are using XAMPP with default settings, this will be something named htdocs in which your project will live. In this case:
C:\xampp\htdocs\mywebsite
This will be the path to store all the website files, including PHP scripts, HTML documents, CSS stylesheets, and images.
3. Writing Basic HTML Structure
First of all, create an index.html file. We can call it the skeleton of an online page. In HTML, you’re supposed to provide the skeleton of your content: headers, paragraphs, links. Here’s a general template for an HTML page:
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>My First PHP Website</title>
<link rel=”stylesheet” href=”styles.css”>
</head>
<body>
<header>
<h1>Welcome to My PHP Website</h1>
</header>
<section>
<p>This is a simple page built using PHP and HTML.</p>
</section>
<footer>
<p>© 2024 My Website</p>
</footer>
</body>
</html>
4. Introduce PHP to Display Dynamic Content
Once you have the rough HTML written, you can start adding PHP. PHP allows you to include dynamic content in your pages, such as today’s date or information loaded from a database. To do so, instead of naming a file index.html, you would name it index.php:
<?php
echo “<p>Today’s date is: “. date(“Y-m-d”). “</p>”;
?>
The date() function returns the current date dynamically in the above example, which is one of the simplest uses of PHP.
5. Form Handling and Working with Data
PHP is frequently used to process user input. Let’s build a simple contact form:
<form action=”contact.php” method=”POST”>
<label for=”name”>Name:</label>
Input: <input type=”text” name=”name” id=”name”>
<label for=”email”>Email:</label>
<input type=”email” name=”email” id=”email”>
<button type=”submit”>SUBMIT</button>
</form>
In contact.php you process the form data:
php
Copy code
<?php
if ($_SERVER[“REQUEST_METHOD”] == “POST”) {
$name = htmlspecialchars($_POST[‘name’]);
$email = htmlspecialchars($_POST[’email’]);
echo “Thank you, “. $name. “. We will contact you at “. $email. “.”;
}
?>
The following example shows example form handling, input sanitization, and dynamic content creation.
6. Design Your Website
Once your functionalities in PHP are ready, you can style your web-page using CSS. You create a file named styles.css and you link that with the html or php file. Example:
css
Copy code
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
}
header {
background-color: #333;
color: white; padding: 20px;
text-align: center; } footer { text-align: center; padding: 10px; background-color: #333; color: white; }
7. Testing and Debugging
Testing, after coding is important, to ensure that the website works as it is supposed to. Check the execution of PHP scripts, correct data submission of forms. Use debugging by print statement using echo or PHP var_dump() to investigate variables and how to trace data in your site.
Conclusion
A PHP website for beginners would entail mastering the basic principles of web development; structuring your content with HTML, adding dynamic behaviour courtesy of PHP, and making it look a bit better courtesy of CSS. In a few steps you are able to make a simple dynamic website, probably serving grounds for more complex projects.
Leave a Reply